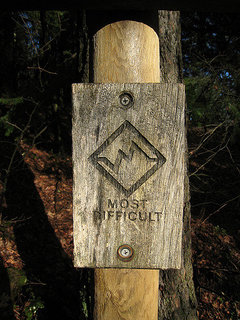
A constraint, or activity, is an artificial challenge during an exercise. I have discussed some of them in the past. Some constraints like No If, Cyclomatic Complexity One or Only Void Methods are easy to follow but make it hard to write your usual code. To have more challenge chose constraints that work against the assignment, e.g. use an algorithmic challenge together with Only Void Methods. Algorithms are often functional in nature but void methods are no functions. Win!
To make things more interesting, constraints can be combined. For example, Object and Functional Calisthenics are constraints that combine several rules. When creating combined constraints, it is important to make sure the constraints work together. There is no point in forcing a functional style with No Void Methods and an object oriented style with Only Void Methods at the same time. When Martin Klose and I combined the Brutal Coding Constraints we spent around 20 hours experimenting and fine tuning them. By the way, these Brutal Coding Constraints are probably one of the most challenging.
When the list of constraints gets long, it is easy to make a mistake and forget to follow one or another. In these situations you need a reviewer, e.g. Coding Dojo facilitator, pair programming partner or static code analysis tool, who checks for violations of constraints.
Harder: Changing Requirements
Another way to spice up an exercise is to introduce requirement changes. This is particularly useful for groups, e.g. Coding Dojos, when participants do not know which requirement is going to change. For the usual Coderetreat exercise Game of Life, several interesting changes have been proposed, e.g. Hex Life, Vampire Cells and the toughest constraint (Wormholes) by Adrian Bolboaca.
I witnessed Martin Klose taking this to the next level: In his exercise Wind of Change, he puts on a tie (because he is the product owner now) and keeps changing the requirements every few minutes. This is a lot of fun and adds some time pressure as well.
Requirement changes is useful to verify a design, usually used in double sessions on software design during Coderetreats. When you are on your own, as soon as you finished the exercise, you think of changes to the requirements and how they would affect your current design.
Harder: Algorithmic Challenges
Algorithmic challenges vary from easy to impossible. Project Euler even has a difficulty rating on each exercise. Often algorithmic challenges are based on mathematics, which makes them not useful for people with less academic background. Also, as soon as you found a solution, the exercises get boring. Using additional constraints can make them fun again, but that would be different exercises then.
I have seen senior developers being more interested in algorithms than XP practises like Pair Programming or TDD. Algorithms are a perfect way to "lure" them into attending Coding Dojos. After a few dojos, people understand the value of practise and will agree to do basic katas with focus on TDD.
If you need a challenge, go for an algorithmic kata and chose a difficult exercise like Potter, Searching or one from Project Euler above number 20.
Harder: Try to be Faster
I do not like to apply time pressure during exercises, because people get sloppy when under pressure. On the other hand, this is what needs to be trained to not get sloppy. Houssam Fakih explains this with a short video (where three people throw basket balls. One is a beginner and fails from time to time, one is experienced and wins repeatedly and one, a "master", is doing the same, but much faster.) Houssam's suggestion is to do the same, but try to be faster. I did that once because I wanted to squeeze an one hour life refactoring demo into a 45 minutes presentation slot. It was hard work, exactly what I wanted.
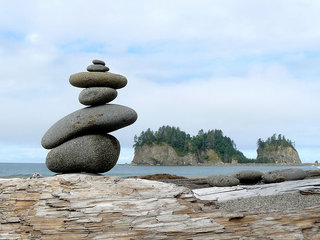
When using constraints and other techniques I describe above, it is easy to go over the top. The exercises become too difficult and working on it is frustrating and eventually we stop doing it. While this might be OK for yourself, it must not happen when working with a group. Exercises like Brutal Coding Constraints are very difficult and not - I repeat - not suitable for a general audience. People tend to overestimate their skill and get frustrated easily.
When facilitating a Coding Dojo, I want to stay in control of the difficulty of the exercise for all participants. I aim for easier, simpler exercises and keep the difficult ones for myself. In rare cases, when I meet very skilled people, I assign them individual constraints, because I know them and I am confident that they will handle. I also make sure everyone understands that it is difficult what they want to do.
Making it Easier: Simpler Assignments
Start with a simple problem. There is always a smaller assignment, The smallest kata I know is FizzBuzz, it is just a single function. There is nothing wrong with FizzBuzz and its friends. I do it from time to time when I explore a new language or try different constraints (or when it is very late and I feel tired). Some function katas like Prime Factors are small too, but algorithmic in nature, so stay away from them. These katas are called FizzBuzz or Function Katas.
Easier: Use Well Known Problems
Solving a programming assignment includes many steps: e.g. understanding the problem, finding a solution, implementing the solution, testing it, etc. The assignment is easier if we get rid of some of these steps. If we use a well known problem, e.g. a ticket machine or a game everyone knows, we already know what is expected.
Easier: Clarify/Understand the Problem
Often the problem with an exercise is that people do not understand the problem. We are eager to get into the code, but we need to understand the assignment first: Take time to analyse the problem you want to solve. Google it. If it is a game like Tic-Tac-Toe, Minesweeper or Pac-Man, find an online version and play for a while. Draw some sketches or diagrams of what needs to be done. Create an list of initial acceptance criteria. To find them, you have to think about the problem. In Coding Dojos I ask people to spend the first ten minutes on creating a test list. This forces them into thinking about the problem.
If you practise with a partner, which I highly recommend, try Adi's pair programming game Solution Seeker. Solution Seeker makes you find at least three different solutions to your problem before you are allowed to implement one of them. This forces you to think hard about the problem and different options to solve it.
As a facilitator, make sure you fully understand the problem so you can answer any question about it. Give more explanations and discuss the problem from different angles. Provide posters or handouts of the problem for participants for later reference.

Repeating the exactly same exercise is considered boring, but it helps. You will understand the assignment better after working on it once. After implementing it several times, maybe even in different programming languages, more and more aspects of the implementation are known and you can go deeper. (This why we run Game of Life in a Coderetreat six times. We do not want to fight with a new problem each session.) This is especially true for hard problems or if you are not satisfied with your process or final solution.
Easier: Focus on One Thing
After repeating the same exercise one or more times, the problem is sufficiently known and you can shift your focus to something else. Using a well known problem is also a way to focus on one thing, in this case you do not focus on solving the problem. There are exercises that isolate different aspects of development: For example, if I want to focus on finding test cases and designing unit tests, I go for the Gilded Rose. If I want to practice refactoring, I do Tennis or Yatzy. Both code bases contain ugly code which is more or less fully covered with tests, making it safe to refactor. There are exercises isolating other things, like incremental development, emergent design, SOLID principles, etc.
Koans belong into this category. Koans are series of little exercises, starting with basic things and building on each other to move to more advanced topics. They are useful to learn programming languages. They contain a list of failing test cases, where tiny pieces of code have to be filled in to make them pass. The idea is not only applicable to programming language constructs. For example I have created Unit Testing Koans to teach xUnit assertions and life cycle to junior developers.
All these exercises require prepared code. For example Gilded Rose is available in 26 languages, including lesser common ones like ABAP and PL/SQL. Trivia even contains COBOL and VB6 - which is very suitable for a legacy code exercise. Obviously prepared code limits the number of languages which can be used. If you want to practice in a new language like Elixir, Elm or Swift, you might need to port the code base first. Although, if the new language is trending, chances are high that someone already ported it.
Easier: Prepared (Helper) Code
Prepared code is useful in many situations, especially outside the core of the practise. Even code snippets or cheat sheets help. For example when I run the Data Munging exercise with focus on functional programming in Java, I show participants code snippets how to read the text file. File IO is not related to the exercise and I want them to spend time working with Lambda expressions and Stream.
Prepared code allows us to focus on one thing, but we need to understand the code first. Unfortunately this adds extra complexity. Unless you want to practice working with unreadable code, prepared code must be simple and super clean. Try to make it more expressive, maybe even verbose, than your usual code and use very descriptive names. Describe the code in the assignment. If there are more methods or classes, visualise their relations. For example in my Test Double exercise, I added a simple diagram of the prepared classes and their collaborators.
Easier: Guide Step by Step
Alex Bolboaca once told me that as facilitator of an exercise it is most important to manage participants' frustration. When I notice that most of the participants are unable to move forward, I take control of the group and guide them step by step. I am not giving them answers, but moderate the necessary process. Maybe we need to discuss the problem before hand on a white board. Or we discuss potential solutions up front. To get an initial test list, I keep asking how we will verify our product until we have a reasonable number of test cases. Sometimes I switch to Mob Programming where the whole group works on the assignment together and I am able to support them best (a.k.a. micro manage).
Conclusion
There are many options to make coding exercises easier or more difficult. I recommend starting easy. There is no point in hurting yourself or others. ;-)
Thanks to Kacper Kuczek and Damian Lukasik for discussing this with me.