
I like programming languages and my learning approach is driven by curiosity and fun. Today I will describe my "standard", step by step way to get into a new language. I will assume you are an experienced developer, able to code in Java or C# who understands basic programming principles. I believe this approach is not suitable for programming newbies. I never use all these steps and sometimes change their order - like starting with the last one. Feel free to reorder or skip any steps not adding knowledge or fun.
1) Get an overview of core language features.
I start reading Wikipedia about the programming language I want to dive into. I am looking for the core features, used concepts and paradigms in the language. Code examples of these features provide a first idea of the language's syntax. The idea is not to know everything, just to be able to write some code. Writing code is the fun part, not reading. The more languages you know the easier and faster this step is. When learning Go one hour on Wikipedia during commute was enough. On the other hand for TypeScript I spent several hours reading the language reference (Handbook). For C# I skipped this step as I had seen most of the language features while facilitating Mob Programming sessions. You are done with this step when you have some idea what the language can do.
2) Figure out the usual setup and get it working.
Most programming languages come with their own ecosystem of runtimes, documentation, dependency and packaging mechanisms, testing frameworks, editors and other tools. When starting with a new language I try to use its typical tooling. Or at least I look for a decent IDE plugin to keep some level of comfort and productivity. Often this is painful and full of compromise. E.g. when working with Scheme I should have used Emacs but started with a basic editor and used VS Code in the end. For Go I needed 2 to 3 hours to set up the command line tools and VS Code integration. For C it took me 2 hours to compile and run a sample test alone - due to incompatible architecture binaries, sigh. I am still staying away from make tools due to the additional complexity. Eventually I will have to work through them if I want to use high level IDEs like Eclipse or CLion. If your interest in the language is purely educational, a simple editor might be enough to get started, like I used for Forth. You are done when you are able to edit, compile, test and run a Hello World application, e.g. in Windows Assembly.
3) Port small pieces of code (from a similar language if possible).
This helped me when learning C. I knew its basic features and had figured out how to compile and run a single file. And then I ported some small (refactoring) code katas. Porting code katas was easier than coding them because the solution and its code were already there and all I needed to deal with was syntax. If there is a similar language to start from, e.g. Java to C#, Java to PHP, C++ to C, even Java to C, then some of the syntax is proper right from the start. There was a lot of Google and StackOverflow involved and I managed to convert a small kata in around one hour. In the end I had ported several code katas to C. Emily Bache keeps inventing fun and interesting refactoring katas, which always need ports to other languages. For example I have ported her Parrot-Refactoring-Kata to TypeScript, Go and recently C. I have also contributed a Scheme version, but that was after I had learnt the language. You are done when you have contributed at least two ports of small refactoring katas.
4) Work through koans of the language.
Koans are an effective way to learn new programming languages. Programming language koans are a progressive sequence of little exercises, starting with basic things and building on each other to move to more advanced topics. The goal is to learn the language and core libraries. Usually the exercises contain failing test cases, where tiny pieces of code have to be filled in to make them pass. (I have used this idea to teach unit testing in Java and PHP as well as Python and C#. Porting the koans from JUnit to xUnit also helped me to get into C# - see the paragraph on porting small pieces of code.) Koans are awesome and I use them regularly. I worked through some Python koans, two third of Kotlin koans and all C Koans. The koans vary in size. While Kotlin contained 6 lessons, Python had 39 and JavaScript even 78. Working all these 78 exercises took me more than three full work days in total. You are done when you have completed (almost) all of the koans for the new language.
Ninety-Nine Problems
In case there are no koans for your language, look for "Ninety-Nine Problems". The idea is based on Werner Hett's P-99: Ninety-Nine Prolog Problems. These are little problems with different levels of difficulty. Sample solutions are available in Java, Scala, Haskell, Kotlin, F#, OCaml and probably others.
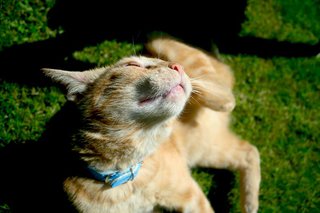
This is the most obvious step. I like to watch recorded presentations, during my commute. Sometimes some extra (passive) information helps me understand a language, or even this specific weird feature. Depending on time and interest this can be a few talks, or whole months of commute.
6) TDD some code katas from scratch.
Now is the time to write some code from scratch. I recommend starting with simple exercises. There is no point in getting frustrated right at the start. Manage the difficulty of your exercises: Use simple katas like FizzBuzz, Prime Factors, Roman Numerals or Word Wrap to get started. For example I used FizzBuzz to practice some XSLT and Prime Factors when revisiting old languages I used to know many years ago - like BASIC. I reuse these katas - and know their solutions - I just want to try them in a new language. Later I move to more complicated assignments like Bowling, Minesweeper (when I revisited Assembly after 20 years), Bank OCR (when I studied Scheme) or Conway's Game of Life. You are done when you have implemented a few code katas from scratch including tests.
7) Write more code, e.g. tackle a larger code base.
There is no other way of getting deeper into a programming language than using it. This calls for a larger side project. It can be a complex code kata, a little video game like Pong or Tron (Nibbles) - including graphics of course - or whatever comes to your mind. As I am fond of Scheme, I have build several Scheme interpreters in new languages. You could even implement your own unit testing framework, which is also recommended by Kent Beck as an exercise to get into a new language. (And I did that for Pascal, Assembly and Scheme.) Depending on the time invested into the previous steps, a side project includes more or less trial and error. If there is too much hassle, I stop and go back to previous steps to learn more about the basics. There is no point in being stuck. For example when studying Go, I went for the side project after 3 hours of researching the language, which was too early. So I spent some more time on theory and then continued working on my idea. My usual learning side projects take around 15 to 20 hours - or that is the time when I lose interest. You are done when you have worked on a larger code base for at least 15 to 20 hours.
8) At last get *all* the details.
Now that I am familiar with the basics of the language and its ecosystem, it is time to dive deeper. After some month of experimenting and hands-on practice - steps 2, 3, 4, 6 and 7 all involve writing code - I am drawn back to theory. I like to balance my learning between theory, experiments and practice. To conclude learning a new language I might study a classic book about that language. It should cover the language and its features completely and I read it from cover to cover. At that time I am already familiar with many parts of the language, reading progress is fast. I am interested in the all the details, the bits and pieces I did not encounter during my experiments. Language specifications are usually dry and perfectly suited for this step as well as classic titles like the "Pickaxe book", K&R and SICP (although SICP is so much more than a Scheme book...) You are done when you read a classic book on the new language from cover to cover.
Conclusion
I like programming languages and learning new ones is adventurous and fun. I try to learn a new language every year. Not all learning goes deep. Not all languages stick. Unless you are working on real projects in all of these languages at the same time, it is natural to forget some details. And that is perfectly fine. It is all about incorporating new paradigms and widening your perspective. So keep learning!