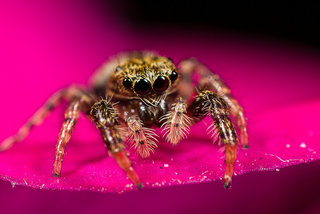
Numbers
The
rt.jar
and other JARs located in the lib
folder of the JRE contain many classes. For each new release I take a look at the new (public) classes. The list of new classes complements the list of new language features. Java 8 adds more than 1000 public classes, mainly in the packages javafx.*
. Without JavaFX there are 251 added classes, similar to Java 7.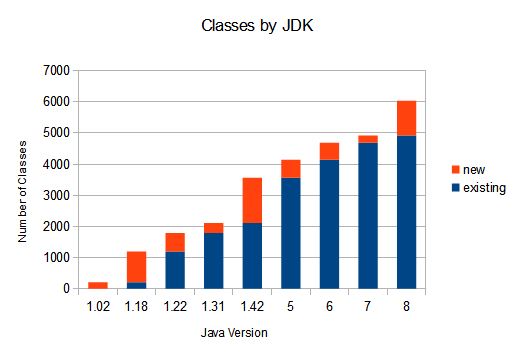
Lambdas and Streams
The major library changes are to support Lambda Expressions and the related Stream API. The new
java.util.function
package contains all types of possible function types.java.lang.FunctionalInterface java.lang.invoke.LambdaConversionException java.lang.invoke.LambdaMetafactory java.lang.invoke.MethodHandleInfo java.lang.invoke.SerializedLambda java.util.function. BiFunction Function Predicate ToDoubleBiFunction DoubleFunction BiPredicate ToIntBiFunction IntFunction DoublePredicate ToLongBiFunction LongFunction IntPredicate LongPredicate BinaryOperator DoubleToIntFunction DoubleBinaryOperator DoubleToLongFunction Supplier IntBinaryOperator IntToDoubleFunction BooleanSupplier LongBinaryOperator IntToLongFunction DoubleSupplier LongToDoubleFunction IntSupplier Consumer LongToIntFunction LongSupplier BiConsumer ToDoubleFunction DoubleConsumer ToIntFunction UnaryOperator IntConsumer ToLongFunction DoubleUnaryOperator LongConsumer IntUnaryOperator ObjDoubleConsumer LongUnaryOperator ObjIntConsumer ObjLongConsumerThe Streams are in the package
java.util.stream
,Stream Stream$Builder StreamSupport BaseStream DoubleStream DoubleStream$Builder IntStream IntStream$Builder LongStream LongStream$Builder Collector Collector$Characteristics Collectorsas well as some additions to
java.util
for splitting and collecting.java.util.DoubleSummaryStatistics java.util.IntSummaryStatistics java.util.LongSummaryStatistics java.util.Spliterator java.util.Spliterator$OfDouble java.util.Spliterator$OfInt java.util.Spliterator$OfLong java.util.Spliterator$OfPrimitive java.util.Spliterators java.util.Spliterators$AbstractDoubleSpliterator java.util.Spliterators$AbstractIntSpliterator java.util.Spliterators$AbstractLongSpliterator java.util.Spliterators$AbstractSpliterator java.util.SplittableRandom java.util.StringJoinerJava 8 Date and Time API
The next big change is the new date and time API. It contains a lot of new classes to model and use dates and time in your code.
java.time.Clock ...format.DateTimeFormatter java.time.DateTimeException ...format.DateTimeFormatterBuilder java.time.DayOfWeek ...format.DateTimeParseException java.time.Duration ...format.DecimalStyle java.time.Instant ...format.FormatStyle java.time.LocalDate ...format.ResolverStyle java.time.LocalDateTime ...format.SignStyle java.time.LocalTime ...format.TextStyle java.time.Month java.time.MonthDay ...temporal.ChronoField java.time.OffsetDateTime ...temporal.ChronoUnit java.time.OffsetTime ...temporal.IsoFields java.time.Period ...temporal.JulianFields java.time.Year ...temporal.Temporal java.time.YearMonth ...temporal.TemporalAccessor java.time.ZonedDateTime ...temporal.TemporalAdjuster java.time.ZoneId ...temporal.TemporalAdjusters java.time.ZoneOffset ...temporal.TemporalAmount ...temporal.TemporalField ...chrono.AbstractChronology ...temporal.TemporalQueries ...chrono.ChronoLocalDate ...temporal.TemporalQuery ...chrono.ChronoLocalDateTime ...temporal.TemporalUnit ...chrono.Chronology ...temporal.UnsupportedTemporalTypeException ...chrono.ChronoPeriod ...temporal.ValueRange ...chrono.ChronoZonedDateTime ...temporal.WeekFields ...chrono.Era ...chrono.HijrahChronology ...zone.ZoneOffsetTransition ...chrono.HijrahDate ...zone.ZoneOffsetTransitionRule ...chrono.HijrahEra ...zone.ZoneOffsetTransitionRule$TimeDefinition ...chrono.IsoChronology ...zone.ZoneRules ...chrono.IsoEra ...zone.ZoneRulesException ...chrono.JapaneseChronology ...zone.ZoneRulesProvider ...chrono.JapaneseDate ...chrono.JapaneseEra ...chrono.MinguoChronology ...chrono.MinguoDate ...chrono.MinguoEra ...chrono.ThaiBuddhistChronology ...chrono.ThaiBuddhistDate ...chrono.ThaiBuddhistEraAnd Other Classes
There are several new classes all over the place. The most notable are:
- Reflection for Type Annotations.
- Finally a Base 64 encoder and decoder.
Optional
.CompletableFuture
andStampedLock
.
java.lang.annotation.Native java.io.UncheckedIOException java.lang.annotation.Repeatable java.net.URLPermission java.lang.reflect.AnnotatedArrayType java.lang.reflect.AnnotatedParameterizedType java.sql.DriverAction java.lang.reflect.AnnotatedType java.sql.JDBCType java.lang.reflect.AnnotatedTypeVariable java.sql.SQLType java.lang.reflect.AnnotatedWildcardType java.lang.reflect.Executable java.util.Base64 java.lang.reflect.MalformedParametersException java.util.Base64$Decoder java.lang.reflect.Parameter java.util.Base64$Encoder java.security.DomainLoadStoreParameter java.util.Calendar$Builder java.security.KeyStore$Entry$Attribute java.util.Locale$FilteringMode java.security.PKCS12Attribute java.util.Locale$LanguageRange java.security.spec.DSAGenParameterSpec java.util.Optional java.security.cert.CertPathChecker java.util.OptionalDouble java.security.cert.PKIXRevocationChecker java.util.OptionalInt java.security.cert.PKIXRevocationChecker$Option java.util.OptionalLong java.util.spi.CalendarDataProvider java.util.PrimitiveIterator java.util.spi.CalendarNameProvider java.util.PrimitiveIterator$OfDouble java.util.spi.ResourceBundleControlProvider java.util.PrimitiveIterator$OfInt java.util.PrimitiveIterator$OfLong java.util.concurrent.atomic.DoubleAccumulator java.util.concurrent.atomic.DoubleAdder java.util.concurrent.atomic.LongAccumulator java.util.concurrent.atomic.LongAdder java.util.concurrent.CompletableFuture java.util.concurrent.CompletableFuture$AsynchronousCompletionTask java.util.concurrent.CompletionException java.util.concurrent.CompletionStage java.util.concurrent.ConcurrentHashMap$KeySetView java.util.concurrent.CountedCompleter java.util.concurrent.locks.StampedLockAdditional changes cover additions to UI capabilities - JavaFX is now part of the JRE. The Java Language Model has been updated (
javax.lang.model
) and Rhino has been replaced by Nashorn (package jdk.nashorn
). There are more changes, e.g. stronger security algorithms, but these do not show up as new classes.Summary
Java 8 brings big changes for the language, finally adding closures to Java. This is as ground breaking as Java 5's Generics. Lambdas and Streams will change the way we write Java and allow a more functional style in our code.
You can download the complete list of all classes available in Java 8. Each class name is annotated with [release] showing the release it first appeared, e.g.
java.lang.annotation.Annotation [5]
. Package access classes are marked by a lowercase p
, e.g. java.lang.CharacterName [7p]
.