As I am the code cop here, I tend to torture colleagues with forbidden references ;-) So one made this little modification to a comic from xkcd by Randall Munroe. I came across it recently while organising some project folders... So here it is:

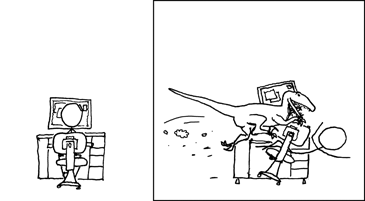
Fanatic About Code Quality Since 2004
'\u2192'
or you found it in the tables of the Unicode Database. char
or int
value. All characters of the Unicode version 4.2.0 up to \u1FFFF
are covered except CJK Ideographs. For each Unicode block, e.g. Basic Latin (\u0000..\u007F
) or Aegean Numbers (\u10100..\u1013F
), there is a separate interface with the block's name defining all code-points defined in this block. First you need to import the blocks, e.g. import unicode.AegeanNumbers
. Then you can use the constants in your code like here:Character.charCount(BasicLatin.DIGIT_NINE)) // 1(And yes, I know, interfaces are a poor place for constants. They should only be used to model a behaviour of a class. See the AvoidConstantsInterface rule. But I was young and needed the money... ;-)
Character.getNumericValue(BasicLatin.DIGIT_NINE)) // 9
Character.charCount(NumberForms.ROMAN_NUMERAL_FIVE_HUNDRED)) // 1
Character.getNumericValue(NumberForms.ROMAN_NUMERAL_FIVE_HUNDRED)) // 500
Character.charCount(AegeanNumbers.NUMBER_EIGHT)) // 2
Character.getNumericValue(AegeanNumbers.NUMBER_EIGHT)) // 8
\u10000
, called code-points, you need Java 1.5 or newer. UCC is Open Source under the GPL license.
Demo
class' main
method:// get a horoscop instanceIn case you want to add or change something, there are the following packages:
TextHoroscop horoscop = new TextHoroscop();
// set your desired planet position calculation algorithm
horoscop.setPlanet(new PlanetAA0());
// set your desired house system calculation algorithm
// may be anything from the at.kugel.zodiac.house package.
horoscop.setHouse(new HousePlacidus());
// set your user data time value
horoscop.setTime(1, 12, 1953, 20);
// set your user data location value
horoscop.setLocationDegree(-16 - 17.0/60, 48 + 4.0/60);
// calculate the values
horoscop.calcValues();
// do something with the data, e.g. output raw data
System.out.println(horoscop.toString());
TextHoroscop
class, which uses the house system and planet calculation classes to calculate the astro-chart data.util
contains some independent calculation utility functions and classes.planet
contains the different planet position calculation algorithms. This package is only dependent on the util package. All planet position algorithms are subclasses of PlanetBasic
which itself implements PlanetInt
. New and more accurate planet position algorithms must implement PlanetInt
and may wish to extend PlanetBasic
to inherit common functionality, but are not forced to do so. There is only PlanetAA0
available at the moment, which is not very accurate.house
contains the different house systems. All house system calculation algorithms are subclasses of HouseBasic
which itself implements HouseInt
.Demo
class in 2004 and fixing a bug using time zones in 2005 (thanks to Rastislav Szabo for reporting it). Today I reformatted the code and fixed some warnings. That's it folks.